Author |
Topic: Direct3D 11 example in BB4W (Read 1370 times) |
|
rtr2
Guest
|
 |
Direct3D 11 example in BB4W
« Thread started on: Jul 31st, 2015, 01:31am » |
|
OK, the reality is not quite as exciting as the subject line suggests: all the program below does is to clear the window to a uniform dark blue colour. But it is genuine Direct3D 11 code, and it could form the basis of greater things. To my absolute astonishment it ran first time (once typos had been corrected):
Code: REM Minimal Direct3D 11 application in BBC BASIC for Windows
REM (C) R. T. Russell, 31-Jul-2015, http://www.bbcbasic.co.uk
MODE 8
REM!WC Declare constants:
NULL = 0
S_OK = 0
DXGI_FORMAT_R8G8B8A8_UNORM = 28
DXGI_USAGE_RENDER_TARGET_OUTPUT = 1 << (1 + 4)
D3D_DRIVER_TYPE_HARDWARE = 1
D3D11_SDK_VERSION = 7
IID_ID3D11Texture2D = FN_guid("{6f15aaf2-d208-4e89-9ab4-489535d34f9c}")
REM Declare structures:
DIM DXGI_RATIONAL{Numerator%, Denominator%}
DIM DXGI_MODE_DESC{Width%, Height%, RefreshRate{} = DXGI_RATIONAL{}, \
\ Format%, ScanlineOrdering%, Scaling%}
DIM DXGI_SAMPLE_DESC{Count%, Quality%}
DIM DXGI_SWAP_CHAIN_DESC{BufferDesc{} = DXGI_MODE_DESC{}, \
\ SampleDesc{} = DXGI_SAMPLE_DESC{}, \
\ BufferUsage%, \
\ BufferCount%, \
\ OutputWindow%, \
\ Windowed%, \
\ SwapEffect%, \
\ Flags%}
DIM D3D11_VIEWPORT{TopLeftX%,TopLeftY%,Width%,Height%,MinDepth%,MaxDepth%}
REM Declare interfaces:
DIM IDXGISwapChain{QueryInterface%,AddRef%,Release%,SetPrivateData%, \
\ SetPrivateDataInterface%,GetPrivateData%,GetParent%,GetDevice%,Present%, \
\ GetBuffer%,SetFullscreenState%,GetFullscreenState%,GetDesc%,ResizeBuffers%, \
\ ResizeTarget%,GetContainingOutput%,GetFrameStatistics%,GetLastPresentCount%}
DIM ID3D11Texture2D{QueryInterface%,AddRef%,Release%,GetDevice%, \
\ GetPrivateData%,SetPrivateData%,SetPrivateDataInterface%,GetType%, \
\ SetEvictionPriority%,GetEvictionPriority%,GetDesc%}
DIM ID3D11Device{QueryInterface%,AddRef%,Release%,CreateBuffer%, \
\ CreateTexture1D%,CreateTexture2D%,CreateTexture3D%,CreateShaderResourceView%, \
\ CreateUnorderedAccessView%,CreateRenderTargetView%,CreateDepthStencilView%, \
\ CreateInputLayout%,CreateVertexShader%,CreateGeometryShader%, \
\ CreateGeometryShaderWithStreamOutput%,CreatePixelShader%,CreateHullShader%, \
\ CreateDomainShader%,CreateComputeShader%,CreateClassLinkage%,CreateBlendState%, \
\ CreateDepthStencilState%,CreateRasterizerState%,CreateSamplerState%, \
\ CreateQuery%,CreatePredicate%,CreateCounter%,CreateDeferredContext%, \
\ OpenSharedResource%,CheckFormatSupport%,CheckMultisampleQualityLevels%, \
\ CheckCounterInfo%,CheckCounter%,CheckFeatureSupport%,GetPrivateData%, \
\ SetPrivateData%,SetPrivateDataInterface%,GetFeatureLevel%,GetCreationFlags%, \
\ GetDeviceRemovedReason%,GetImmediateContext%,SetExceptionMode%,GetExceptionMode%}
DIM ID3D11DeviceContext{QueryInterface%,AddRef%,Release%,GetDevice%, \
\ GetPrivateData%,SetPrivateData%,SetPrivateDataInterface%,VSSetConstantBuffers%, \
\ PSSetShaderResources%,PSSetShader%,PSSetSamplers%,VSSetShader%, \
\ DrawIndexed%,Draw%,Map%,Unmap%,PSSetConstantBuffers%,IASetInputLayout%, \
\ IASetVertexBuffers%,IASetIndexBuffer%,DrawIndexedInstanced%,DrawInstanced%, \
\ GSSetConstantBuffers%,GSSetShader%,IASetPrimitiveTopology%,VSSetShaderResources%, \
\ VSSetSamplers%,Begin%,End%,GetData%,SetPredication%,GSSetShaderResources%, \
\ GSSetSamplers%,OMSetRenderTargets%,OMSetRenderTargetsAndUnorderedAccessViews%, \
\ OMSetBlendState%,OMSetDepthStencilState%,SOSetTargets%,DrawAuto%, \
\ DrawIndexedInstancedIndirect%,DrawInstancedIndirect%,Dispatch%, \
\ DispatchIndirect%,RSSetState%,RSSetViewports%,RSSetScissorRects%, \
\ CopySubresourceRegion%,CopyResource%,UpdateSubresource%,CopyStructureCount%, \
\ ClearRenderTargetView%,ClearUnorderedAccessViewUint%,ClearUnorderedAccessViewFloat%, \
\ ClearDepthStencilView%,GenerateMips%,SetResourceMinLOD%,GetResourceMinLOD%, \
\ ResolveSubresource%,ExecuteCommandList%,HSSetShaderResources%,HSSetShader%, \
\ HSSetSamplers%,HSSetConstantBuffers%,DSSetShaderResources%,DSSetShader%, \
\ DSSetSamplers%,DSSetConstantBuffers%,CSSetShaderResources%,CSSetUnorderedAccessViews%, \
\ CSSetShader%,CSSetSamplers%,CSSetConstantBuffers%,VSGetConstantBuffers%, \
\ PSGetShaderResources%,PSGetShader%,PSGetSamplers%,VSGetShader%, \
\ PSGetConstantBuffers%,IAGetInputLayout%,IAGetVertexBuffers%,IAGetIndexBuffer%, \
\ GSGetConstantBuffers%,GSGetShader%,IAGetPrimitiveTopology%,VSGetShaderResources%, \
\ VSGetSamplers%,GetPredication%,GSGetShaderResources%,GSGetSamplers%, \
\ OMGetRenderTargets%,OMGetRenderTargetsAndUnorderedAccessViews%, \
\ OMGetBlendState%,OMGetDepthStencilState%,SOGetTargets%,RSGetState%, \
\ RSGetViewports%,RSGetScissorRects%,HSGetShaderResources%,HSGetShader%, \
\ HSGetSamplers%,HSGetConstantBuffers%,DSGetShaderResources%,DSGetShader%, \
\ DSGetSamplers%,DSGetConstantBuffers%,CSGetShaderResources%,CSGetUnorderedAccessViews%, \
\ CSGetShader%,CSGetSamplers%,CSGetConstantBuffers%,ClearState%,Flush%, \
\ GetType%,GetContextFlags%,FinishCommandList%}
REM: Initialise:
SYS "LoadLibrary","D3D11.DLL" TO d3d11%
IF d3d11% = 0 ERROR 0, "DirectX 11 is not installed"
SYS "GetProcAddress", d3d11%, "D3D11CreateDeviceAndSwapChain" TO \
\ `D3D11CreateDeviceAndSwapChain`
REM Create the swap chain:
DIM sd{} = DXGI_SWAP_CHAIN_DESC{}
sd.BufferCount% = 1
sd.BufferDesc.Width% = 640
sd.BufferDesc.Height% = 512
sd.BufferDesc.Format% = DXGI_FORMAT_R8G8B8A8_UNORM
sd.BufferDesc.RefreshRate.Numerator% = 60
sd.BufferDesc.RefreshRate.Denominator% = 1
sd.BufferUsage% = DXGI_USAGE_RENDER_TARGET_OUTPUT
sd.OutputWindow% = @hwnd%
sd.SampleDesc.Count% = 1
sd.SampleDesc.Quality% = 0
sd.Windowed% = 1
SYS `D3D11CreateDeviceAndSwapChain`, NULL, D3D_DRIVER_TYPE_HARDWARE, \
\ NULL, 0, NULL, 0, D3D11_SDK_VERSION, sd{}, ^pSwapChain%, \
\ ^pd3dDevice%, NULL, ^pImmediateContext% TO result%
IF result% <> S_OK ERROR 100, "CreateDeviceAndSwapChain failed"
!(^IDXGISwapChain{}+4) = !pSwapChain%
!(^ID3D11Device{}+4) = !pd3dDevice%
!(^ID3D11DeviceContext{}+4) = !pImmediateContext%
REM Create a render target view:
SYS IDXGISwapChain.GetBuffer%, pSwapChain%, 0, IID_ID3D11Texture2D, \
\ ^pBackBuffer% TO result%
IF result% <> S_OK ERROR 100, "IDXGISwapChain::GetBuffer failed"
!(^ID3D11Texture2D{}+4) = !pBackBuffer%
SYS ID3D11Device.CreateRenderTargetView%, pd3dDevice%, pBackBuffer%, NULL, \
\ ^pRenderTargetView% TO result%
IF result% <> S_OK ERROR 100, "ID3D11Device::CreateRenderTargetView failed"
SYS ID3D11Texture2D.Release%, pBackBuffer%
SYS ID3D11DeviceContext.OMSetRenderTargets%, pImmediateContext%, \
\ 1, ^pRenderTargetView%, NULL
DIM vp{} = D3D11_VIEWPORT{} : REM All members are floats
vp.Width% = FN_f4(sd.BufferDesc.Width%)
vp.Height% = FN_f4(sd.BufferDesc.Height%)
vp.MinDepth% = 0
vp.MaxDepth% = FN_f4(1.0)
vp.TopLeftX% = 0
vp.TopLeftY% = 0
SYS ID3D11DeviceContext.RSSetViewports%, pImmediateContext%, 1, vp{}
REM Render:
DIM ClearColor%(3)
ClearColor%() = 0, FN_f4(0.125), FN_f4(0.6), FN_f4(1.0) : REM RGBA
SYS ID3D11DeviceContext.ClearRenderTargetView%, pImmediateContext%, \
\ pRenderTargetView%, ^ClearColor%(0)
SYS IDXGISwapChain.Present%, pSwapChain%, 0, 0
END
DEF FN_f4(a#)
LOCAL A% : PRIVATE F%
IF F% = 0 THEN
SYS "LoadLibrary", "OLEAUT32.DLL" TO F%
SYS "GetProcAddress", F%, "VarR4FromR8" TO F%
ENDIF
a# *= 1.0#
SYS F%,!^a#,!(^a#+4),^A%
=A%
DEF FN_guid(a$)
LOCAL C%, M% : PRIVATE O%
DIM C% 30, M% LOCAL 2*LENa$+2
C% = (C%+15) AND -16 : M% = (M%+1) AND -2
IF O% = 0 THEN
SYS "LoadLibrary", "OLE32.DLL" TO O%
SYS "GetProcAddress", O%, "CLSIDFromString" TO O%
ENDIF
SYS "MultiByteToWideChar", 0, 0, a$, -1, M%, LENa$+1
SYS O%, M%, C%
= C% Richard.
|
« Last Edit: Jul 31st, 2015, 09:02am by rtr2 » |
Logged
|
|
|
|
rtr2
Guest
|
 |
Re: Direct3D 11 example in BB4W
« Reply #1 on: Jul 31st, 2015, 09:24am » |
|
on Jul 31st, 2015, 01:31am, g4bau wrote:it is genuine Direct3D 11 code, and it could form the basis of greater things. |
|
What I propose to do, if there is sufficient interest, is to publish a series of Direct3D 11 articles (based directly on the Microsoft tutorials, as this code was) on the BB4W Wiki. I realise that some people might prefer Direct3D 12, but very few users currently have that and it's difficult to find good tutorials to plagiarise adapt!
Thoughts?
Richard.
|
« Last Edit: Jul 31st, 2015, 09:27am by rtr2 » |
Logged
|
|
|
|
Torro
New Member
member is offline


Posts: 25
|
 |
Re: Direct3D 11 example in BB4W
« Reply #2 on: Jul 31st, 2015, 10:18am » |
|
Has the Rubicon been crossed 
Yes that would be good
Re Directx12 Documentation https://msdn.microsoft.com/en-us/library/windows/desktop/dn899121(v=vs.85).aspx
samples [urlhttps://msdn.microsoft.com/en-us/library/windows/desktop/mt186620(v=vs.85).aspx][/url]
Nvidia currently only have native driver support for there latest graphics cards . I have the previous chipset which at present is not support for dx12 feature boo. and is coming soon. In any case having support would beat many others to the punch.
Im also interested in process you take Richard to bridge the link between the DLL and a basic library/code
Also was that utility to extract header reading the DLL directly or a C++ header file source code?
|
|
Logged
|
|
|
|
rtr2
Guest
|
 |
Re: Direct3D 11 example in BB4W
« Reply #3 on: Jul 31st, 2015, 2:47pm » |
|
on Jul 31st, 2015, 10:18am, Torro wrote:Re Directx12 Documentation |
|
I know it's documented, but there are not (as yet) any Microsoft tutorials - starting with something simple and working up to more complicated tasks - similar to those for DirectX 11.
Quote:I'm also interested in process you take Richard to bridge the link between the DLL and a basic library/code |
|
I'm translating the C code in the tutorials to BASIC. It's largely a 'mechanical' process but not one that it's practical to automate, so quite tedious and time-consuming. I've yet to discover what issues the later tutorials may raise.
Quote:Also was that utility to extract header reading the DLL directly or a C++ header file source code? |
|
It scans D3D9.H (header file) and I've now written a similar one to scan D3D11.H.
Richard.
|
|
Logged
|
|
|
|
Michael Hutton
Developer
member is offline


Gender: 
Posts: 248
|
 |
Re: Direct3D 11 example in BB4W
« Reply #4 on: Jul 31st, 2015, 2:56pm » |
|
A lot of this has already been done in 2010.
Code:
REM ****************************************************************
REM
REM Create a DirectX11 Device
REM
REM ****************************************************************
DEF PROC_Init_D3D11(hwnd%)
INSTALL @lib$ + "DirectX11\Functions\DLL"
INSTALL @lib$ + "DirectX11\Functions\Helper"
CALL @lib$ + "DirectX11\Constants"
CALL @lib$ + "DirectX11\Structures"
CALL @lib$ + "DirectX11\Interfaces"
PROC_Helper_AssembleFNf
REM Get the Dlls and the functions we need
D3D11DLL% = FN_DLL_LoadDLL("D3D11.DLL")
D3D11CreateDeviceAndSwapChain% = FN_DLL_GetFunctionAddress(D3D11DLL%, "D3D11CreateDeviceAndSwapChain")
D3DX11DLL% = FN_DLL_LoadDLL(FN_GetD3DX11DLL)
D3DX11CompileFromFile% = FN_DLL_GetFunctionAddress(D3DX11DLL%, "D3DX11CompileFromFileA")
REM Create our swap chain description{}
LOCAL scd{}
DIM scd{} = DXGI_SWAP_CHAIN_DESC{}
REM Fill out its members
scd.BufferCount% = 1
scd.BufferDesc.Width% = SCREENWIDTH
scd.BufferDesc.Height% = SCREENHEIGHT
scd.BufferDesc.Format% = DXGI_R8G8B8A8_UNORM
scd.BufferDesc.RefreshRate.Numerator% = 60
scd.BufferDesc.RefreshRate.Denominator% = 1
scd.BufferUsage% = DXGI_USAGE_RENDER_TARGET_OUTPUT
scd.OutputWindow% = hwnd%
scd.SampleDesc.Count% = 4
scd.SampleDesc.Quality% = 0
scd.Windowed% = _TRUE
scd.Flags% = DXGI_SWAP_CHAIN_FLAG_ALLOW_MODE_SWITCH
REM Create the device
SYS D3D11CreateDeviceAndSwapChain%, 0, \
\ D3D_DRIVER_TYPE_HARDWARE, \
\ 0, \
\ 0, \
\ 0, \
\ 0, \
\ D3D11_SDK_VERSION , \
\ scd{}, \
\ ^SwapChain%, \
\ ^Device%, \
\ 0, \
\ ^DeviceContext% TO R%
IF R% PROC_D3DERR(R%) ELSE IF V% PRINT"Device Created."
PROC_Helper_CreateInterface(IDevice{}, ID3D11Device{}, Device%)
PROC_Helper_CreateInterface(ISwapChain{}, IDXGISwapChain{}, SwapChain%)
PROC_Helper_CreateInterface(IDeviceContext{}, ID3D11DeviceContext{}, DeviceContext%)
REM Get the address of the back buffer
SYS ISwapChain.GetBuffer%, SwapChain%, 0, IID_ID3D11Texture2D{}, ^pBackBuffer% TO R%
IF R% THEN ERROR 100,"Error : swapchain->GetBuffer : "+STR$~R%
REM Use the BackBufferAddress to create the Render Target
SYS IDevice.CreateRenderTargetView%, Device%, pBackBuffer%, 0, ^BackBuffer% TO R%
IF R% THEN ERROR 100,"Error : Couldn't Use the BackBufferAddress to create the Render Target"
REM Release the BackBuffer pointer
SYS !(!pBackBuffer% + 8), pBackBuffer%
REM OMSetRenderTargets : Returns Void
SYS IDeviceContext.OMSetRenderTargets%, DeviceContext%, 1, ^BackBuffer%, 0
REM Set the viewport
DIM vp{} = D3D11_VIEWPORT{}
vp.Width% = FNf(SCREENWIDTH)
vp.Height% = FNf(SCREENHEIGHT)
vp.TopLeftX% = 0
vp.TopLeftY% = 0
SYS IDeviceContext.RSSetViewports%, DeviceContext%, 1, vp{}
ENDPROC
REM ****************************************************************
REM
REM Closes DirextX11 and releases all the Objects created
REM
REM ****************************************************************
DEF PROC_Close_D3D11(D%)
PRINT'
REM switch to windowed mode
SwapChain% += 0
IF SwapChain% THEN
SYS ISwapChain.SetFullscreenState%, SwapChain%, 0, 0
ENDIF
REM Release Roger!
REM Release all our objects
IF FN_Helper_Release(VertexShader%) = 0 IF V% PRINT"VertexShader released."
IF FN_Helper_Release(PixelShader%) = 0 IF V% PRINT"PixelShader released."
IF FN_Helper_Release(BackBuffer%) = 0 IF V% PRINT"BackBuffer released."
IF FN_Helper_Release(SwapChain%) = 0 IF V% PRINT"Swapchain released."
IF FN_Helper_Release(Device%) = 0 IF V% PRINT"Device released."
IF FN_Helper_Release(DeviceContext%) = 0 IF V% PRINT"DeviceContext released."
REM Release our dlls
IF FN_DLL_FreeDLL(D3D11DLL%) IF V% THEN PRINT"D3D11.DLL Freed."
IF FN_DLL_FreeDLL(D3DX11DLL%) IF V% THEN PRINT"D3DX11.DLL Freed."
ENDPROC
DEF FN_Helper_Release(RETURN O%)
LOCAL R%
O% += 0 : IF O% THEN SYS !(!O%+8), O% TO R%
=R%
REM ****************************************************************
REM
REM DirextX11 Error handling
REM
REM ****************************************************************
DEF PROC_D3DERR(E%)
D3DERR_INVALIDCALL = FN_MAKE_D3DHRESULT(2156)
D3DERR_WASSTILLDRAWING = FN_MAKE_D3DHRESULT(540)
ENDPROC
DEF FN_MAKE_D3DHRESULT(C%)
=FN_MAKE_HRESULT(1, &876, C%)
DEF FN_MAKE_D3D10_HRESULT(C%)
=FN_MAKE_HRESULT(1, &879, C%)
DEF FN_MAKE_D3D11_HRESULT(C%)
=FN_MAKE_HRESULT(1, &87C, C%)
DEF FN_MAKE_HRESULT(S%, F%, C%)
=(S%<<31) OR (F%<<16) OR C%
I have all the libraries needed at the moment. Of course, they could probably be updated.
If there is sufficient interest I could upload them.
Michael.
|
|
|
|
rtr2
Guest
|
 |
Re: Direct3D 11 example in BB4W
« Reply #5 on: Jul 31st, 2015, 4:50pm » |
|
on Jul 31st, 2015, 2:56pm, Michael Hutton wrote:A lot of this has already been done in 2010. |
|
You said in another thread: "I only have a 'starter file', basically getting a device going" so it would seem that in fact you didn't even get as far as the degree of functionality in the code that I posted this morning. That may not do much, but at least there's something to see!
What I am specifically doing is translating the Microsoft tutorials (there are others out there, but they seem to take bigger steps and in some cases you need to pay a subscription before you get to the end!). When I eventually put the code on the Wiki I want to be able to cross-reference those tutorials, where there is much more detail.
In any case I want to understand this stuff to a degree that I can provide support to other users, and there's no better way than doing it myself.
So thanks, but no thanks.
Richard.
|
|
Logged
|
|
|
|
rtr2
Guest
|
 |
Re: Direct3D 11 example in BB4W
« Reply #6 on: Jul 31st, 2015, 9:16pm » |
|
on Jul 31st, 2015, 09:24am, g4bau wrote:What I propose to do, if there is sufficient interest, is to publish a series of Direct3D 11 articles based directly on the Microsoft tutorials |
|
Making good progress: today I've managed to get Tutorial 2 running (admittedly it didn't work first time). The code is too long to list here (and needs to be tidied up before public consumption) but as evidence here is a rendered triangle. I know it's not rocket science, but it takes nearly 300 lines of code to achieve it in DirectX 11!
Edit: I should add that I've departed from the tutorial code slightly in order to avoid the D3DX issue. I'm using the D3DCompiler DLL, which comes as standard with Windows 8.1/10 and which is in any case legally redistributable.
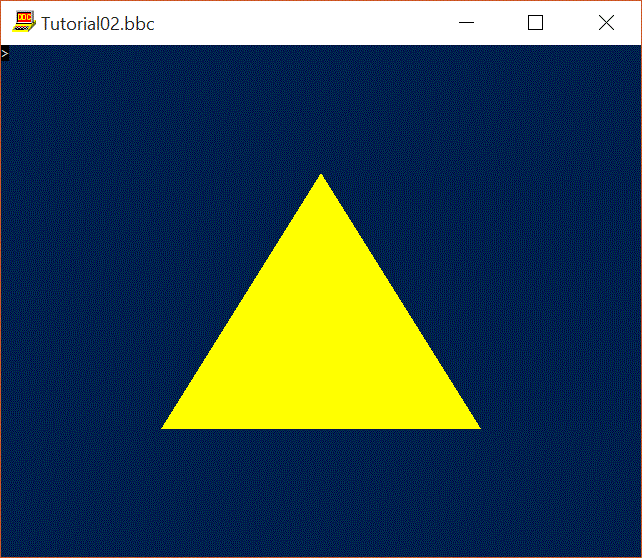
Richard.
|
« Last Edit: Jul 31st, 2015, 10:33pm by rtr2 » |
Logged
|
|
|
|
rtr2
Guest
|
 |
Re: Direct3D 11 example in BB4W
« Reply #7 on: Aug 1st, 2015, 9:24pm » |
|
|
|
Logged
|
|
|
|
Torro
New Member
member is offline


Posts: 25
|
 |
Re: Direct3D 11 example in BB4W
« Reply #8 on: Aug 2nd, 2015, 10:00pm » |
|
Are these directx11 hlsl tutorials usefull? http://rastertek.com/tutdx11.html
|
|
Logged
|
|
|
|
Torro
New Member
member is offline


Posts: 25
|
 |
Re: Direct3D 11 example in BB4W
« Reply #9 on: Aug 4th, 2015, 10:12am » |
|
Have the direct x 11 tutorials code been uiploaded some where?
The Directx11 header files? Library?
|
|
Logged
|
|
|
|
DDRM
Administrator
member is offline


Gender: 
Posts: 321
|
 |
Re: Direct3D 11 example in BB4W
« Reply #10 on: Aug 5th, 2015, 2:49pm » |
|
Richard has now posted the D3D11 tutorials, and a link to a zip with all the supporting files, on the wiki:
http://bb4w.wikispaces.com/Direct3D+11+Tutorials
Best wishes,
D
|
|
Logged
|
|
|
|
DDRM
Administrator
member is offline


Gender: 
Posts: 321
|
 |
Re: Direct3D 11 example in BB4W
« Reply #11 on: Aug 6th, 2015, 3:34pm » |
|
...and now the 7th tutorial, and updated library etc files, are available at the wiki.
D
|
|
Logged
|
|
|
|
Torro
New Member
member is offline


Posts: 25
|
 |
Re: Direct3D 11 example in BB4W
« Reply #12 on: Aug 10th, 2015, 5:53pm » |
|
windows 10 come s with directx 11.3 a higher level version of directx12
|
|
Logged
|
|
|
|
|