Author |
Topic: Displaying a histogram in a dialog box (Read 1072 times) |
|
g3nrw
Junior Member
member is offline


Posts: 74
|
 |
Displaying a histogram in a dialog box
« Thread started on: Jul 29th, 2014, 09:02am » |
|
Here is a very simple program that generates a histogram:
Code:
MODE 8
GCOL 131
GCOL 12
CLG
MOVE 200,300
FOR n% = 0 TO 18
x% = n% * 50
y% = n% * 20
RECTANGLE FILL x%,30,35,y%
NEXT
Can someone point me to reference(s) that explain how to plot this in a dialog box?
-- Ian
|
|
Logged
|
|
|
|
rtr
Guest
|
 |
Re: Displaying a histogram in a dialog box
« Reply #1 on: Jul 29th, 2014, 12:00pm » |
|
on Jul 29th, 2014, 09:02am, g3nrw wrote:Can someone point me to reference(s) that explain how to plot this in a dialog box? |
|
There are various ways it might be done, but one simple method would be to save your plot to a BMP file (OSCLI "GSAVE....) and then display that bitmap in a 'picture box' (code to do that is in the BB4W docs). The best place to store the BMP would be the temporary folder @tmp$.
Beware DPI issues, and make sure you test your code at the common DPI values (96, 120, 144 at least).
This technique isn't ideal for a 'live' or 'animated' plot so if that is a requirement you might want to create a custom graphics control using the MULTIWIN library.
This would all be considerably easier in the other dialect of BASIC that I market (as it would have been using GUILIB, but if I keep mentioning that it will become annoying). 
Richard.
|
|
Logged
|
|
|
|
g3nrw
Junior Member
member is offline


Posts: 74
|
 |
Re: Displaying a histogram in a dialog box
« Reply #2 on: Jul 29th, 2014, 12:56pm » |
|
Richard
The histogram will be "animated", being updated each time each time the user opens the dialog box that displays the histogram.
I will investigate the MULTIWIN library.
-- Ian
|
|
Logged
|
|
|
|
rtr
Guest
|
 |
Re: Displaying a histogram in a dialog box
« Reply #3 on: Jul 29th, 2014, 2:03pm » |
|
post removed
|
« Last Edit: Jul 30th, 2014, 08:50am by rtr » |
Logged
|
|
|
|
g3nrw
Junior Member
member is offline


Posts: 74
|
 |
Re: Displaying a histogram in a dialog box
« Reply #4 on: Aug 4th, 2014, 9:37pm » |
|
on Jul 29th, 2014, 12:00pm, Richard Russell wrote:There are various ways it might be done, but one simple method would be to save your plot to a BMP file (OSCLI "GSAVE....) and then display that bitmap in a 'picture box' |
|
I'm getting ever closer. I have written code that creates a simple histogram, then saves the created image as a bitmap file (zzz.bmp), then displays the bitmap in a new dialog box (dlg2).
Code:
REM +++++ A much emasculated DLGDEMO, modified to open a new dialog box
REM to display a histogram
INSTALL @lib$+"WINLIB2"
BS_DEFPUSHBUTTON = &1
CB_ADDSTRING = &143
CB_SETCURSEL = &14E
CBS_DROPDOWNLIST = &3
ES_AUTOHSCROLL = &80
ES_NUMBER = &2000
ES_READ_ONLY = &880
LB_ADDSTRING = &180
LB_GETCURSEL = &188
UDM_SETRANGE = &465
UDS_ALIGNRIGHT = &4
UDS_AUTOBUDDY = &10
UDS_SETBUDDYINT = &2
WS_CHILD = &40000000
WS_GROUP = &20000
WS_VISIBLE = &10000000
dlg%=FN_newdialog("Dialogue box", 20, 20, 160, 128, 8, 32768)
PROC_groupbox(dlg%, "Group box", 0, 4, 4, 152, 96, WS_GROUP)
PROC_pushbutton(dlg%, "OK", 1, 12, 108, 56, 14, WS_GROUP OR BS_DEFPUSHBUTTON)
PROC_pushbutton(dlg%, "Cancel", 2, 92, 108, 56, 14, 0)
REM +++++++++++++++++++++++++++++++++++++++ new code:
INSTALL @lib$+"WINLIB5A"
WS_POPUP = &80000000
dlg2%=FN_newdialog("MY HISTOGRAM", 20, 20, 500, 260, 10, 32768)
PROC_static(dlg2%,"",700,100,100,500,500,&E)
REM +++++++++++++++++++++++++++++++++++++++ end of new code
PROC_showdialog(dlg%)
ON CLOSE PROC_closedialog(dlg%):QUIT
ON ERROR PROC_closedialog(dlg%):PRINT'REPORT$:END
Click%=0
ON SYS Click% = @wparam% : RETURN
REPEAT
WAIT 1
click%=0
SWAP Click%, click%
UNTIL click%=1 OR click%=2 OR !dlg%=0
IF click%=1 THEN
REM +++++++++++++++++++++++++++++++++++++++ new code:
PROC_showdialog(dlg2%)
VDU 23,22,900;650;11,16,16,0
GCOL 135
GCOL 12
CLG
FOR n% = 0 TO 17
x% = n% * 88
y% = n% * 34
RECTANGLE FILL x%+100,150,65,y%
NEXT
file$ = "zzz.bmp"
OSCLI "GSAVE """+file$+""" 100,100,500,500"
LR_LOADFROMFILE = 16
STM_SETIMAGE = 370
SYS "LoadImage", 0, file$, 0, 250, 250, LR_LOADFROMFILE TO hbitmap%
SYS "SendDlgItemMessage", !dlg2%, 700, STM_SETIMAGE, 0, hbitmap%
ELSE
PROC_closedialog(dlg2%)
SYS "DeleteObject", hbitmap%
ENDIF
REM +++++++++++++++++++++++++++++++++++++++ end of new code
END
This works as expected, but the snag is that when I close the dialog box, the computed histogram is still visible in another window that was previously hidden.
Is there a way to run the histogram computation in a "virtual" window without displaying it, or, alternatively, forcing the previously hidden window to close when closing dlg2 ?
-- Ian
|
|
Logged
|
|
|
|
rtr
Guest
|
 |
Re: Displaying a histogram in a dialog box
« Reply #5 on: Aug 4th, 2014, 11:09pm » |
|
on Aug 4th, 2014, 9:37pm, g3nrw wrote:This works as expected, but the snag is that when I close the dialog box, the computed histogram is still visible in another window that was previously hidden |
|
See my earlier post (although it's not clear from your example why you need the histogram to be displayed in a dialogue box at all; an ordinary non-dialogue window looks as if it would suit just as well):
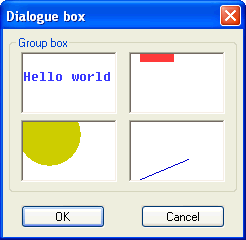
Richard.
|
|
Logged
|
|
|
|
g3nrw
Junior Member
member is offline


Posts: 74
|
 |
Re: Displaying a histogram in a dialog box
« Reply #6 on: Aug 5th, 2014, 05:44am » |
|
Richard
I don't understand your reply:
on Aug 4th, 2014, 11:09pm, Richard Russell wrote:
Which "earlier post"?
Quote: an ordinary non-dialogue window looks as if it would suit just as well): |
|
Don't understand: your 4-window "Hello world"/graphics example *is* in a dialog box.
-- Ian
|
|
Logged
|
|
|
|
rtr
Guest
|
 |
Re: Displaying a histogram in a dialog box
« Reply #7 on: Aug 5th, 2014, 08:34am » |
|
on Aug 5th, 2014, 05:44am, g3nrw wrote: The one in which I announced support for drawing graphics in a custom dialogue control, which I posted yesterday only a few hours before yours. 
This thread triggered me to re-visit that functionality, which was intended to have been provided in GUILIB but, in the absence of that library, has never been supported in BB4W until now.
Quote:Don't understand: your 4-window "Hello world"/graphics example *is* in a dialog box. |
|
The subject line of this thread is "Displaying a histogram in a dialog box". That is exactly the sort of thing the newly-supported functionality, as illustrated by that graphic, allows!
My 'aside' stemmed from the fact that you accompanied your most recent message with a code listing. When I run that program, and click on the OK button, it displays your histogram graphics in what appears to be a regular window - it has no 'dialogue-style' features such as multiple controls or OK/cancel buttons.
Looking at the detail of your code, that window consists of a dialogue box containing a static 'picture box' and nothing else. Therefore it begs the question of why you are using a dialogue box at all, when you could achieve something almost identical with an ordinary 'BBC' window, and in so doing avoid all the complications of drawing graphics and dealing with DPI variation.
Having spent considerable time and effort in providing support for drawing graphics in a dialogue control, it was a bit disconcerting to see that - at least superficially - you don't actually require that functionality at all. 
So do you actually need to "display a histogram in a dialog box" as the thread title implies, or would an ordinary graphics-capable window (created most easily using the MULTIWIN library) suffice?
Richard.
|
|
Logged
|
|
|
|
sveinioslo
Developer
member is offline


Posts: 64
|
 |
Re: Displaying a histogram in a dialog box
« Reply #8 on: Aug 5th, 2014, 11:54pm » |
|
Well Ian, if you want to animate graphics in a dialog, here is one way of doing it. But it so much easier with the new Multiwin library Richard so kindly provided.
Svein
Code: STM_SETIMAGE = 370
SS_BITMAP = &E
BS_DEFPUSHBUTTON = 1
INSTALL @lib$+"WINLIB2"
dlg%=FN_newdialog("Displaying animated histogram in a dialog box", 20, 20, 400, 200, 8, 500)
cx%=380 : cy%=160
PROC_static(dlg%,"",123,10,10,cx%,cy%,SS_BITMAP)
PROC_pushbutton(dlg%, "Start", 1, 240, 180, 56, 14, BS_DEFPUSHBUTTON)
PROC_pushbutton(dlg%, "Cancel", 2, 310, 180, 56, 14, 0)
PROC_showdialog(dlg%)
ON CLOSE PROC_close:QUIT
ON ERROR PROC_close:PRINT'REPORT$:END
DIM rc{l%,t%,r%,b%}
rc.r%=cx% : rc.b%=cy%
SYS "MapDialogRect", !dlg%, rc{}
cx%=rc.r% : cy%=rc.b%
SYS "CreateCompatibleBitmap", @memhdc%, cx%, cy% TO hbitmap%
*REFRESH OFF
Click%=0 : start%=0
ON SYS Click% = @wparam% : RETURN
REPEAT
IF start%=1 THEN
a%+=1 : IF a%>30 THEN a%=1
FOR n% = 0 TO 17
SYS "SelectObject", @memhdc%, hbitmap% TO C%
REM do your graphics here
x% = n% * 64
y% = n% * a%
GCOL RND(15): RECTANGLE FILL x%, 2*@vdu%!212-2*cy%, 32, y%
REM .....................
SYS "SelectObject", @memhdc%, C%
SYS "SendDlgItemMessage", !dlg%, 123, STM_SETIMAGE, 0, hbitmap%
*REFRESH
WAIT 2
NEXT n%
ENDIF
click%=0
SWAP Click%, click%
IF click%=1 THEN start%=1
UNTIL click%=2 OR !dlg%=0
PROC_close : QUIT
DEF PROC_close
PROC_closedialog(dlg%) : SYS "DeleteObject", hbitmap%
ENDPROC
|
|
Logged
|
|
|
|
|