Nested PROC
Post by ScriptBasic on Jan 15th, 2014, 10:32pm
Hi,
I was wondering if I can nest external declared functions and have them return a result?
From the examples I've seen, it looks as if PROC must start the statement.
Example:
MyFunc(ThisFunc(5))
Re: Nested PROC
Post by hellomike on Jan 15th, 2014, 10:49pm
Hi,
Don't confuse PROCs with Functions. PROC don't return a value but funtions do.
For example you can do:
DEF FNMyFunc(x)=x*100
DEF FNThisFunc(x)=x+1
PRINT FNMyFunc(FNThisFunc(5))
END
This will print 600 as result.
The functions are not declared external but this can be done using Libraries.
Hope this helps a bit.
Mike
Re: Nested PROC
Post by admin on Jan 16th, 2014, 08:56am
on Jan 15th, 2014, 10:49pm, hellomike wrote:The functions are not declared external but this can be done using Libraries. |
|
I wonder if the OP is referring to the fact that SYS has only a PROC-like syntax; there isn't an FN-style equivalent to SYS.
As a result you can't nest external (DLL) function calls as conveniently as one might like, and in practice you end up having to wrap the SYS statement in an FN like this:
Code: DEF FN_GetTickCount
LOCAL T%
SYS "GetTickCount" TO T%
= T%
It's a slight inconvenience, and in an ideal world it might be nice to have a functionality such as:
Code: tick% = SYS("GetTickCount") : REM Non-existent syntax
But although it's something I have often thought about I don't expect it's likely ever to be implemented.
Richard.
Re: Nested PROC
Post by ScriptBasic on Jan 16th, 2014, 5:40pm
Thanks Richard for the help with wrapping external API functions to be used in ones code. (why BBC4W has libraries)
Re: Nested PROC
Post by Matt on Jan 16th, 2014, 7:13pm
on Jan 16th, 2014, 08:56am, Richard Russell wrote: Code: tick% = SYS("GetTickCount") : REM Non-existent syntax |
|
I guess if it were necessary, one could always produce a library function which allowed something similar:
Code: result% = FN_SYS("SystemFunction[, par1, par2...]")
where the function returned the value of a the SYS line - although this, in itself would be a lot more complicated that the simple -
Code: SYS "SystemFunction" [, par1, par2...] TO T%
= T%
Matt
Re: Nested PROC
Post by admin on Jan 16th, 2014, 9:39pm
on Jan 16th, 2014, 7:13pm, Matt wrote:I guess if it were necessary, one could always produce a library function which allowed something similar: Code: result% = FN_SYS("SystemFunction[, par1, par2...]") |
|
Sadly not, at least not without major limitations. I assume you are envisaging the use of EVAL to evaluate the parameters, but that's not really acceptable because of the incompatibility with compiling. So, to all intents and purposes, this attempted workaround to the lack of native variadic functions in BB4W doesn't work. :(
Richard.
Re: Nested PROC
Post by JGHarston on Jan 19th, 2014, 3:48pm
SYS has been a command on ARM BBC BASIC for 28 years, and people writing programs have had no problem doing things like:
SYS "thing" TO r%:IF r% THEN....
or wrapping the SYS call in a function, almost universally when doing something in addition to just purely calling the SYS call, eg:
DEFFNreadvar(name$)
SYS "OS_ReadVarVal",name$, blah blah
IF blah blah THEN blah blah etc.
=result
just like, for example, the Windows Registry library.
Re: Nested PROC
Post by ScriptBasic on Jan 19th, 2014, 4:29pm
With Richard's help, I was able to get IUP (Portable User Interface) working with BBC BASIC for Windows. (Win7-64, XP and Wine)
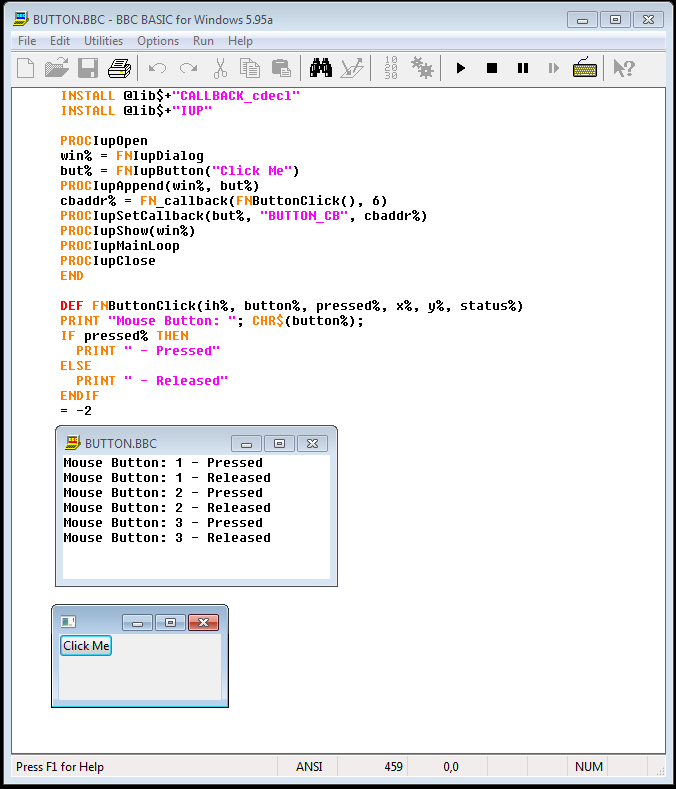
You need this modified by Richard CALLBACK_cdecl.bbc to make the above example work.
BUTTON.BBC
Code:
INSTALL @lib$+"CALLBACK_cdecl"
INSTALL @lib$+"IUP"
PROCIupOpen
win% = FNIupDialog
but% = FNIupButton("Click Me")
PROCIupAppend(win%, but%)
cbaddr% = FN_callback(FNButtonClick(), 6)
PROCIupSetCallback(but%, "BUTTON_CB", cbaddr%)
PROCIupShow(win%)
PROCIupMainLoop
PROCIupClose
END
DEF FNButtonClick(ih%, button%, pressed%, x%, y%, status%)
PRINT "Mouse Button: "; CHR$(button%);
IF pressed% THEN
PRINT " - Pressed"
ELSE
PRINT " - Released"
ENDIF
= -2
IUP.BBC
Code:
REM BBC4W IUP Library
REM By John Spikowski - 1/16/2014
DEF PROCIupOpen
LOCAL gpa$, ll%
gpa$ = "GetProcAddress"
SYS "LoadLibrary", "iup.dll" TO ll%
SYS gpa$, ll%, "IupOpen" TO IupOpen%
SYS gpa$, ll%, "IupDialog" TO IupDialog%
SYS gpa$, ll%, "IupLabel" TO IupLabel%
SYS gpa$, ll%, "IupButton" TO IupButton%
SYS gpa$, ll%, "IupAppend" TO IupAppend%
SYS gpa$, ll%, "IupShow" TO IupShow%
SYS gpa$, ll%, "IupMainLoop" TO IupMainLoop%
SYS gpa$, ll%, "IupClose" TO IupClose%
SYS gpa$, ll%, "IupSetAttributes" TO IupSetAttributes%
SYS gpa$, ll%, "IupSetCallback" TO IupSetCallback%
SYS IupOpen%, 0, 0
ENDPROC
DEF PROCIupClose
SYS IupClose%
ENDPROC
DEF PROCIupShow(iup%)
LOCAL dummy%
SYS FN_syscalln(IupShow%), iup% TO !FN_systo(dummy%)
ENDPROC
DEF PROCIupMainLoop
LOCAL dummy%
SYS FN_syscalln(IupMainLoop%) TO !FN_systo(dummy%)
ENDPROC
DEF PROCIupAppend(target%, src%)
SYS IupAppend%, target%, src%
ENDPROC
DEF FNIupDialog
LOCAL dlg%
SYS IupDialog% TO dlg%
= dlg%
DEF FNIupLabel(title$)
LOCAL lbl%
SYS IupLabel%, title$ TO lbl%
= lbl%
DEF FNIupButton(title$)
LOCAL btn%
SYS IupButton%, title$, "" TO btn%
= btn%
DEF PROCIupSetAttributes(iup%, attribstr$)
SYS IupSetAttributes%, iup%, attribstr$
ENDPROC
DEF PROCIupSetCallback(iup%, cbtype$, cbaddr%)
LOCAL dummy%
cbtype$ += CHR$(0)
REM SYS FN_syscalln(IupSetCallback%), iup%, cbtype$, cbaddr% TO !FN_systo(dummy%)
SYS IupSetCallback%, iup%, cbtype$, cbaddr%
ENDPROC
Re: Nested PROC
Post by admin on Jan 19th, 2014, 5:30pm
on Jan 19th, 2014, 4:29pm, ScriptBasic wrote:With Richard's help, I was able to get IUP (Portable User Interface) working with BBC BASIC for Windows. (Win7-64, XP and Wine) |
|
I can confirm that it works fine in Windows 8.1 too.
Richard.
Re: Nested PROC
Post by admin on Jan 19th, 2014, 9:19pm
on Jan 19th, 2014, 7:33pm, ScriptBasic wrote:Here is an IUP example I did in ScriptBasic |
|
Given that this is a BBC BASIC support forum, can you show us what the code to create that dialog would look like in 'our' BASIC? What advantage (if any) does using IUP offer over the existing BB4W GUI libraries?
Personally I don't like having to depend on a third-party tool over which we have no control; accessing the Windows API directly via BB4W libraries provides the maximum flexibility and reliability, and all the information we could possibly need is available on MSDN.
IUP is described as a 'portable' interface, but given that BB4W only runs under Windows that portability has little if any practical value to the BBC BASIC programmer. And needing to distribute the IUP DLLs would add a large overhead to any compiled application.
Richard.
Re: Nested PROC
Post by admin on Jan 19th, 2014, 9:49pm
on Jan 19th, 2014, 9:24pm, ScriptBasic wrote:IUP based applications look pretty much the same no matter what the binding is. |
|
That may be true, but it's impossible to compare IUP with the existing BB4W GUI libraries without seeing actual code. And, no, that isn't an invitation to list Script Basic code here. 
Quote:You would be foolish to assume that one language can satisfy all needs. |
|
I agree, but learning how to access the Windows GUI directly is by far the best way to acquire skills that are transferrable to another language. And since the necessary DLLs come as standard with Windows it will also result in the most compact executables.
Please try to keep your posts relevant to BBC BASIC for Windows, and not to stray into discussing other languages and operating systems, which are off-topic here.
Richard.
Re: Nested PROC
Post by ScriptBasic on Jan 19th, 2014, 10:04pm
Sorry!
Have removed the off topic content.
Re: Nested PROC
Post by admin on Jan 19th, 2014, 10:20pm
on Jan 19th, 2014, 10:04pm, ScriptBasic wrote:Have removed the off topic content. |
|
It wasn't all off topic, and I concede that it's impossible to discuss the merits of a 'portable' interface without mentioning the existence of other platforms!
But looked at from the perspective of this forum, IUP would need to offer benefits other than portability to attract BB4W users, and indeed its other benefits would have to be substantial to outweigh the disadvantage of distributing the IUP DLLs with every application.
Now, if there was a native Linux version of BBC BASIC, which was substantially compatible with BB4W (apart from the OS-specific features like SYS), then that would put things in an entirely different light. Then IUP could be the solution for genuine cross-platform GUI-based applications written in BBC BASIC.
But, as far as I'm aware, that is currently a distant prospect and it needs somebody other than me to take an interest in it to make it happen.
Richard.
Re: Nested PROC
Post by ScriptBasic on Jan 19th, 2014, 10:28pm
Quote:But looked at from the perspective of this forum, IUP would need to offer benefits other than portability to attract BB4W users, and indeed its other benefits would have to be substantial to outweigh the disadvantage of distributing the IUP DLLs with every application. |
|
When installing VB takes over a GB, adding a few IUP DLLs to your project seems moot. I was thinking of enhancing the BBC BASIC for Windows SQLite3 Address book example and make it GUI based on IUP. How much work would it be to do the same with the GUI tools currently available to BBC4W users? From the GUI demos I've briefly looked at, it seems GUI is a Windows API/SDK style effort.
Re: Nested PROC
Post by admin on Jan 19th, 2014, 11:22pm
on Jan 19th, 2014, 10:28pm, ScriptBasic wrote:When installing VB takes over a GB, adding a few IUP DLLs to your project seems moot. |
|
We're not talking about VB, we're talking about BB4W, and compiled GUI applications can easily be only 100 Kbytes or so. How much might that increase if the IUP DLLs need to be bundled?
I don't buy the argument that as memory and disk sizes increase it doesn't matter if applications run to several megabytes. There are two main reasons: firstly, CPU Level 1 caches are still moderately small, and if you can fit most of your application in the cache there can be significant speed benefits. Secondly, downloading via the internet is still (for most people) sufficiently slow that running applications 'from the cloud' is much more practical if they are small.
Quote: I was thinking of enhancing the BBC BASIC for Windows SQLite3 Address book example and make it GUI based on IUP. How much work would it be to do the same with the GUI tools currently available to BBC4W users? |
|
Not very much, I would expect. As a practical comparison between IUP and the existing BB4W libraries here is a simple button demo using both methods:
IUP version
Code: INSTALL @lib$+"CALLBACK_cdecl"
INSTALL @lib$+"IUP"
PROCIupOpen
win% = FNIupDialog
but% = FNIupButton("Click Me")
PROCIupAppend(win%, but%)
cbaddr% = FN_callback(FNButtonClick(), 6)
PROCIupSetCallback(but%, "BUTTON_CB", cbaddr%)
PROCIupShow(win%)
PROCIupMainLoop
PROCIupClose
END
DEF FNButtonClick(ih%, button%, pressed%, x%, y%, status%)
IF pressed%=0 PRINT "Mouse Button "; CHR$(button%) " clicked"
= -2
WINLIB version
Code: INSTALL @lib$+"WINLIB2"
INSTALL @lib$+"WINLIB5"
win% = FN_newdialog("", 100, 100, 100, 40, 4, 1000)
but% = FN_setproc(PROCButtonClick())
PROC_pushbutton(win%, "Click Me", but%, 0, 0, 40, 16, 0)
PROC_showdialog(win%)
REPEAT
WAIT 1
UNTIL FALSE
DEF PROCButtonClick(wparam%, lparam%)
PRINT "Mouse Button "; &FFFF-wparam% " clicked"
ENDPROC
If anything I would say the version using the existing libraries is a little simpler.
Richard.
Re: Nested PROC
Post by ScriptBasic on Jan 19th, 2014, 11:55pm
That looks great! A victim of not RTFM.
Once again thanks for all the help getting up to speed with BBC4W using a non-supported platform and external tools.
Quote:What gets us into trouble isn't what we don't know; it's what we know for sure that just ain't so!
-- Mark Twain -- |
|
Re: Nested PROC
Post by ScriptBasic on Jan 20th, 2014, 6:56pm
Is there a FN/PROC list anywhere of the Windows GUI API for BBC4W's 13 library files? All I could find in the documentation is a section E. that seems like an introduction more than the libraries user guide. Would the BBC4W wiki be a better place to look?
Update: I found what I was looking for in the library documentation.